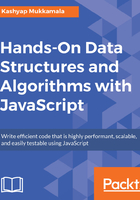
上QQ阅读APP看书,第一时间看更新
Laying out the UI
We will use angular-material to style the app, as it is quick and reliable. To install angular-material, run the following command:
npm install --save @angular/material @angular/animations @angular/cdk
Once angular-material is saved into the application, we can use the Button component provided to create the UI necessary, which will be fairly straightforward. First, import the MatButtonModule that we want to use for this view and then inject the module as the dependency in your main AppModule.
The final form of app.module.ts would be as follows:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { FormsModule } from '@angular/forms';
import { HttpModule } from '@angular/http';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { MatButtonModule } from '@angular/material';
import { AppComponent } from './app.component';
import { RouterModule } from "@angular/router";
import { routes, navigatableComponents } from "./app.routing";
import { Stack } from "./utils/stack";
// main angular module
@NgModule({
declarations: [
AppComponent,
// our components are imported here in the main module
...navigatableComponents
],
imports: [
BrowserModule,
FormsModule,
HttpModule,
// our routes are used here
RouterModule.forRoot(routes),
BrowserAnimationsModule,
// material module
MatButtonModule
],
providers: [
Stack
],
bootstrap: [AppComponent]
})
export class AppModule { }
We will place four buttons at the top to switch between the four states that we have created and then display these states in the router-outlet directive provided by Angular followed by the back button. After all this is done, we will get the following result:
<nav>
<button mat-button
routerLink="/about"
routerLinkActive="active">
About
</button>
<button mat-button
routerLink="/dashboard"
routerLinkActive="active">
Dashboard
</button>
<button mat-button
routerLink="/home"
routerLinkActive="active">
Home
</button>
<button mat-button
routerLink="/profile"
routerLinkActive="active">
Profile
</button>
</nav>
<router-outlet></router-outlet>
<footer>
<button mat-fab (click)="goBack()" >Back</button>
</footer>