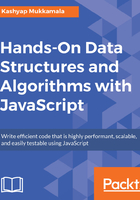
Enabling web worker communications
Now that the app component is set and ready to send messages, the worker needs to be enabled to receive the messages from the main thread. To do that, add the following code to your worker.js file:
init();
function init() {
self.addEventListener('message', function(e) {
var code = e.data;
if(typeof code !== 'string' || code.match(/.*[a-zA-Z]+.*/g)) {
respond('Error! Cannot evaluate complex expressions yet. Please try
again later');
} else {
respond(evaluate(convert(code)));
}
});
}
As you can see, we added the capability of listening for any message that might be sent to the worker and then the worker simply takes that data and applies some basic validation on it before trying to evaluate and return any value for the expression. In our validation, we simply rejected any characters that are alphabetic because we want our users to only provide valid numbers and operators.
Now, start your application using the following command:
npm start
You should see the app come up at localhost:4200. Now, simply enter any code to test your application; for example, enter the following:
var a = 100;
You would see the following error pop up on the screen:

Now, let's get a detailed understanding of the algorithm that is in play. The algorithm will be split into two parts: parsing and evaluation. A step-by-step breakdown of the algorithm would be as follows:
- Converting input expression to a machine-understandable expression.
- Evaluating the postfix expression.
- Returning the expression's value to the parent component.