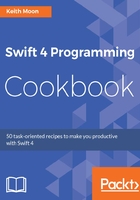
Associated values
Our string-based enum seems perfect for our title information, except that we have a case called other. If the person has a title that we hadn't considered when defining the enum, we can select other, but that doesn't capture what the other title is. We will need to define a whole other property to hold the value of the other title, but there would be nothing to force it to be used when the other case is selected, and nothing to stop it from being used when a different Title case is selected.
Swift enums have a solution for this situation--associated values; we can choose to associate a value with each enum case, allowing us to bind a non-optional string to our other case.
Let's rewrite our Title enum to use an associated value:
enum Title {
case mr
case mrs
case mister
case miss
case dr
case prof
case other(String)
}
We have defined the other case to have an associated value by putting the value's type in brackets after the case declaration. We do not need to add associated values wholesale to every case; other case declarations can have associated values of a different type, or none at all.
Let's look at how we assign an enum case with an associated type:
let mister: Title = .mr
let dame: Title = .other("Dame")
The associated value is declared in brackets after the case, and the compiler enforces that the type matches the type declared in our enum definition. As we declared the other case to have a non-optional string, we are ensuring that a title of other cannot be selected without providing details of what the other title is, and we don't have an unneeded property hanging around when anything else is selected.