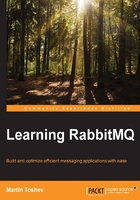
Message router
The following diagram provides an overview of the scenario that we will implement:

Let's say we have a service that triggers an event upon the creation of a new programming seminar, or hackathon, for a given community. We want to send all seminar events to a particular destination receiver and all hackaton events to another destination receiver. Moreover, we want to send messages to the same exchange. For that setup, a topic exchange is a rational choice; one queue will be bound to the topic exchange with the seminar.#
routing key and another queue will be bound with hackaton.#
routing key. The #
character is special and serves as a pattern that matches any character sequence.
We can implement this type of message sending by further extending our Sender
class:
private static final String SEMINAR_QUEUE = "seminar_queue"; private static final String HACKATON_QUEUE = "hackaton_queue"; private static final String TOPIC_EXCHANGE = "topic_exchange"; public void sendEvent(String exchange, String message, String messageKey) { try { channel.exchangeDeclare(TOPIC_EXCHANGE, "topic"); channel.queueDeclare(SEMINAR_QUEUE, false, false, false, null); channel.queueDeclare(HACKATON_QUEUE, false, false, false, null); channel.queueBind(SEMINAR_QUEUE, TOPIC_EXCHANGE, "seminar.#"); channel.queueBind(HACKATON_QUEUE, TOPIC_EXCHANGE, "hackaton.#"); channel.basicPublish(TOPIC_EXCHANGE, messageKey, null, message.getBytes()); } catch (IOException e) { LOGGER.error(e.getMessage(), e); } }
In order to demonstrate event sending, we can use the TopicSenderDemo
class:
public class TopicSenderDemo { private static final String TOPIC_EXCHANGE = "topic_exchange"; public static void sendToTopicExchange() { Sender sender = new Sender(); sender.initialize(); sender.sendEvent(TOPIC_EXCHANGE, "Test message 1.", "seminar.java"); sender.sendEvent(TOPIC_EXCHANGE, "Test message 2.", "seminar.rabbitmq"); sender.sendEvent(TOPIC_EXCHANGE, "Test message 3.", "hackaton.rabbitmq"); sender.destroy(); } public static void main(String[] args) { sendToTopicExchange(); } }