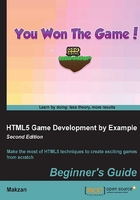
Time for action – placing Ping Pong game elements in the DOM
- We will continue from our jQuery installation example, and open the
index.html
file in a text editor. - Then, we will create the following playground and
game
objects with DIV nodes in the body. There are two paddles and one ball inside the playground, and the playground is placed inside the game:<p id="game"> <p id="playground"> <p class="paddle-hand right"></p> <p class="paddle-hand left"></p> <p id="paddleA" class="paddle"></p> <p id="paddleB" class="paddle"></p> <p id="ball"></p> </p> </p>
- We now have the structure of the
game
object ready, and it is time to apply styles to them. We will add the following styles to thepingpong.css
file:#game { position: relative; width: 400px; height: 200px; } #playground{ background: url(../images/playground.png); background-size: contain; width: 100%; height: 100%; position: absolute; top: 0; left: 0; overflow: hidden; cursor: pointer; } #ball { background: #fbb; position: absolute; width: 20px; height: 20px; left: 150px; top: 100px; border-radius: 10px; }
- Then, we will define the dimensions and positions of the two paddles by appending the following code inside the
pingpong.css
file:.paddle { background-size: contain; top: 70px; position: absolute; width: 30px; height: 70px; } #paddleA { left: 50px; background-image: url(../images/football-player-left.png); } #paddleB { right: 50px; background-image: url(../images/football-player.png); }
- We will continue with the styles in the
pingpong.css
file and definepaddle-hands
, which is the decoration for the paddles:.paddle-hand { background: url(../images/football-player-hand.png) 50% 0 repeat-y; background-size: contain; width: 30px; height: 100%; position: absolute; top: 0; } .left.paddle-hand { left: 50px; } .right.paddle-hand { right: 50px; }
- Now that we are done with the CSS styles, let's move to the
js/pingpong.js
file for JavaScript's logic. We need a function to update the DOM elements of the paddles based on the position data. To do this, we will replace the current code with the following one:(function($){ // data definition var pingpong = { paddleA: { x: 50, y: 100, width: 20, height: 70 }, paddleB: { x: 320, y: 100, width: 20, height: 70 }, }; // view rendering function renderPaddles() { $("#paddleB").css("top", pingpong.paddleB.y); $("#paddleA").css("top", pingpong.paddleA.y); } renderPaddles(); })(jQuery);
- Now, we will test the setup in a browser. Open the
index.html
file in a browser; we should see a screen similar to the one shown in the following screenshot:
What just happened?
Let's take a look at the HTML code that we just used. The HTML page contains header, footer information, and a DIV element with the ID, game
. The game
node contains a child named playground
, which in turn contains three children—two paddles and the ball.
We often start the HTML5 game development by preparing a well-structured HTML hierarchy. The HTML hierarchy helps us to group similar game objects (which are some DIV elements) together. It is a little like grouping assets into a movie clip in Adobe Flash, if you have ever made animations with it. We may also consider it as layers of game objects for us to select and style them easily.
Using jQuery
The jQuery command often contains two major parts: selection and modification. Selection uses CSS selector syntax to select all matched elements in the web page. Modification actions modify the selected elements, such as adding or removing children or style. Using jQuery often means chaining selection and modification actions together.
For example, the following code selects all elements with the box
class and sets the CSS properties:
$(".box").css({"top":"100px","left":"200px"});
Understanding basic jQuery selectors
jQuery is about selecting elements and performing actions on them. We need a method to select our required elements in the entire DOM tree. jQuery borrows the selectors from CSS. The selector provides a set of patterns to match elements. The following table lists the most common and useful selectors that we will use in this book:

Understanding the jQuery CSS function
The jQuery css
function works to get and set the CSS properties of the selected elements. This is known as getting and setting pattern where many jQuery functions follow.
Here is a general definition of how to use the css
function:
.css(propertyName) .css(propertyName, value)
The css
function accepts several types of arguments as listed in the following table:

Manipulating game elements in DOM with jQuery
We initialized the paddles' game elements with jQuery. We will do an experiment on how we should use jQuery to place the game elements.
Understanding the behavior of absolute position
When a DOM node is set to be at the absolute
position, the left and top properties can be treated as a coordinate. We can treat the left/top properties into X/Y coordinates with Y positive pointing down. The following graphs show the relationship. The left side is the actual CSS value, and the right side is the coordinate system in our mind when programming the game:

By default, the left and top properties refer to the top-left edge of the web page. This reference point is different when any parent of this DOM node has a position
style set explicitly to relative
or absolute
. The reference point of the left and top properties becomes the top-left edge of that parent.
This is why we need to set the game with a relative position and all game elements inside it with an absolute position. The following code snippet from our example shows the position values of the elements:
#game{ position: relative; } #playground, #ball, #paddle { position: absolute; }
Declaring global variables in a better way
Global variables are variables that can be accessed globally in the entire document. Any variable that is declared outside any function is a global variable. For instance, in the following example code snippet, a
and b
are global variables, while c
is a local variable that only exists inside the function:
var a = 0; var b = "xyz"; function something(){ var c = 1; }
Since global variables are available in the entire document, they may increase the chance of variable name conflicts if we integrate different JavaScript libraries into the same web page. As good practice, we should minimize the use of global variables.
In the preceding Time for action section, we have an object to store the game data. Instead of just putting this object in the global scope, we created an object named pingpong
and put the data inside it.
Moreover, when we put all our logic into a self-executing function, as we discussed in the last section, we make the game's data object locally inside the function scope.
Note
Declaring variables without var
puts the variables in the global scope even when they are defined inside a function scope. So, we always declare variables with var
.
Pop quiz
Q1. Which jQuery selector is to be used if you want to select all header elements?
$("#header")
$(".header")
$("header")
$(header)