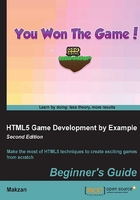
Time for action – saving the circle position
- Open the
untangle.data.js
file in the text editor. - Add the following
circle
object definition code in the JavaScript file:untangleGame.Circle = function(x,y,radius){ this.x = x; this.y = y; this.radius = radius; }
- Now we need an array to store the circles' positions. Add a new array to the
untangleGame
object:untangleGame.circles = [];
- While drawing every circle in the Canvas, we save the position of the circle in the
circles
array. Add the following line before calling thedrawCircle
function, inside thecreateRandomCircles
function:untangleGame.circles.push(new untangleGame.Circle(x,y,circleRadius));
- After the steps, we should have the following code in the
untangle.data.js
file:if (untangleGame === undefined) { var untangleGame = {}; } untangleGame.circles = []; untangleGame.Circle = function(x,y,radius){ this.x = x; this.y = y; this.radius = radius; }; untangleGame.createRandomCircles = function(width, height) { // randomly draw 5 circles var circlesCount = 5; var circleRadius = 10; for (var i=0;i<circlesCount;i++) { var x = Math.random()*width; var y = Math.random()*height; untangleGame.circles.push(new untangleGame.Circle(x,y,circleRadius)); untangleGame.drawCircle(x, y, circleRadius); } };
- Now we can test the code in the web browser. There is no visual difference between this code and the last example when drawing random circles in the Canvas. This is because we are saving the circles but have not changed any code that affects the appearance. We just make sure it looks the same and there are no new errors.
What just happened?
We saved the position and radius of each circle. This is because Canvas drawing is an immediate mode. We cannot directly access the object drawn in the Canvas because there is no such information. All lines and shapes are drawn on the Canvas as pixels and we cannot access the lines or shapes as inpidual objects. Imagine that we are drawing on a real canvas. We cannot just move a house in an oil painting, and in the same way we cannot directly manipulate any drawn items in the canvas
element.
Defining a basic class definition in JavaScript
We can use object-oriented programming in JavaScript. We can define some object structures for our use. The Circle
object provides a data structure for us to easily store a collection of x and y positions and the radii.
After defining the Circle
object, we can create a new Circle
instance with an x, y, and radius value using the following code:
var circle1 = new Circle(100, 200, 10);
Note
For more detailed usage on object-oriented programming in JavaScript, please check out the Mozilla Developer Center at the following link:
https://developer.mozilla.org/en/Introduction_to_Object-Oriented_JavaScript
Have a go hero
We have drawn several circles randomly on the Canvas. They are in the same style and of the same size. How about we randomly draw the size of the circles? And fill the circles with different colors? Try modifying the code and then play with the drawing API.